To learn about all the methods and properties of any HTML element, consult the Mozilla Developer Network page on Elements.
Click on any element to find information on its available properties and methods.
For example, if I want to learn how to modify classes attached to an element, I can look through this document and see the classList or className properties, as seen in the screenshot below:
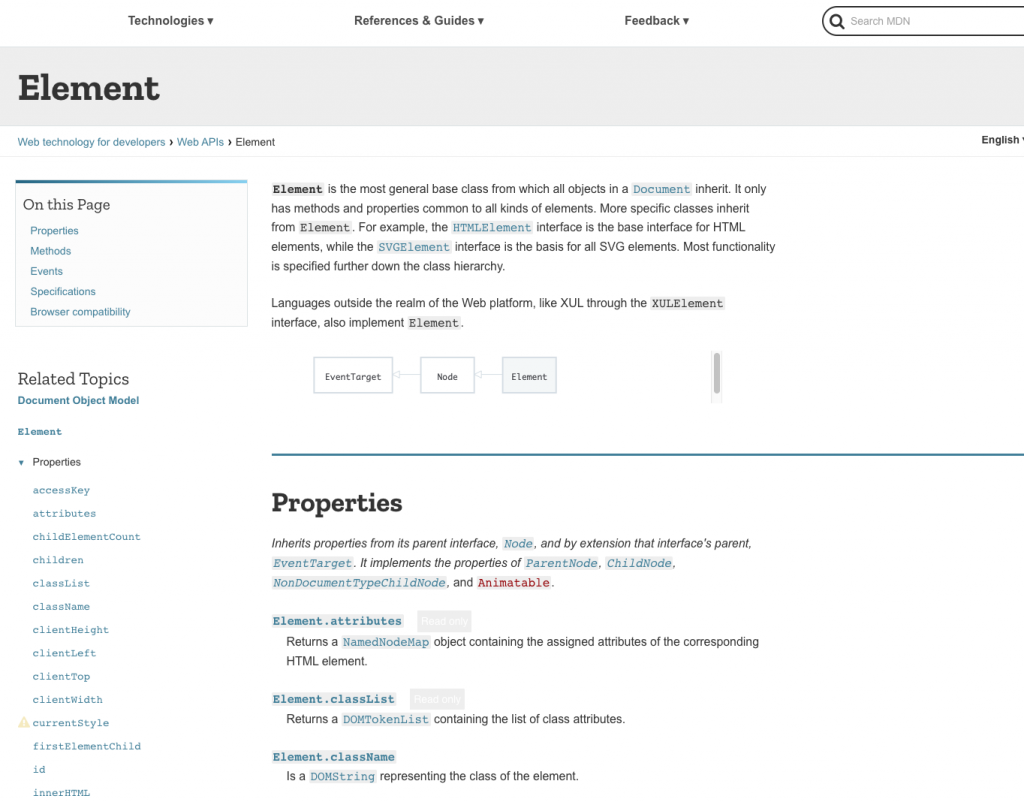
If I click on Element.classList, I learn that the classList has six methods:
- add
- remove
- toggle
- item
- contains
- replace
Also included is information about what form the methods’ respective arguments take.
All in all, MDN is an excellent resource: it’s concise and very informative.
So to make a simple JS event handler that adds or removes a class on the body when another element is clicked, we’d do something like this:
const btn1 = document.querySelector('.button1');
btn1.addEventListener('click', function(){
document.body.classList.toggle('something-is-active');
});
Here, we’re selecting a button by a classname, telling it to listen for a click, and when clicked to toggle a class on the body of the document.
We could use add or remove methods here. Toggle, however, contains conditional logic: it will add the class if the class is not already present, or remove it if it is already present.
By adding a class to the body of the document, we now can target anything on the page with a descendent selector that applies only when the class is present: .something-is-active .specific-element-you-want-to-style.